Python Introduction
Python is an interpreted, object-oriented, high-level programming language with dynamic semantics. Its high-level built-in data structures, combined with dynamic typing and dynamic binding, make it very attractive for Rapid Application Development.
-from python.org
Guido Van Rossum developed the python programming language as a successor of the ABC programming language in 1991. It is named after the “Monty python's Flying Circus” comedy series. It is widely used in reputed companies like Google, Dropbox, Netflix, NASA, NSA, Bit torrent, and all over the world. It’s used in software development and data science. It’s good for starting compared to other programming languages because of its simplicity. PyCharm is an open-source IDE for python development.
Oops Concepts
Oops, stands for Object-Oriented Programming.
Class
A class in python is a “blueprint” for creating an object. It helps in building data and functions together to create a new instance.
Syntax:
#Define class
Class class_name:
Var1 = data1
Var2 = data2
Def fun1(self):
return(“Welcome to python”)
#Call class
C = class_name()
c.fun1()
[Output]
“Welcome to python”
Class example (Delivery system):
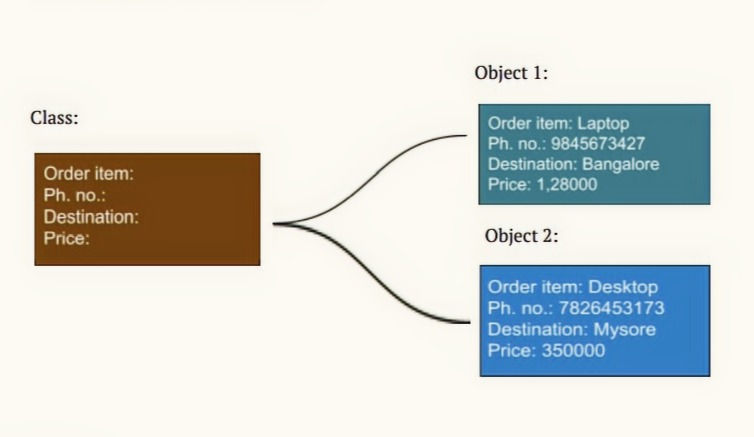
2. Object
An object is an instance of a class. It contains all the properties of the class. One class can have more than one object.
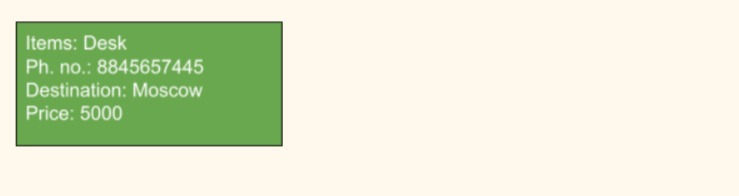
3. Method
A method is a function of an object. Method access data within and may also alter an object state.
Example:
#Class Method
Class delivery:
Def cal_price(items):
return(price_data(items))
4. Inheritance
Inheritance is the fundamental property in python. It helps to inherit the features of one class into another class. The properties inherited from the class are called a parent or superclass. The properties inherited from the class are called child or sub-class.
Example:
For example:
#Parent Class
Class shape:
Def set_color(self):
return(“Red”)
#Child Class
Class circle(shape):
Def set_name(self):
return(“Circle one”)
C = circle()
c.set_color()
[Output]
“Red”
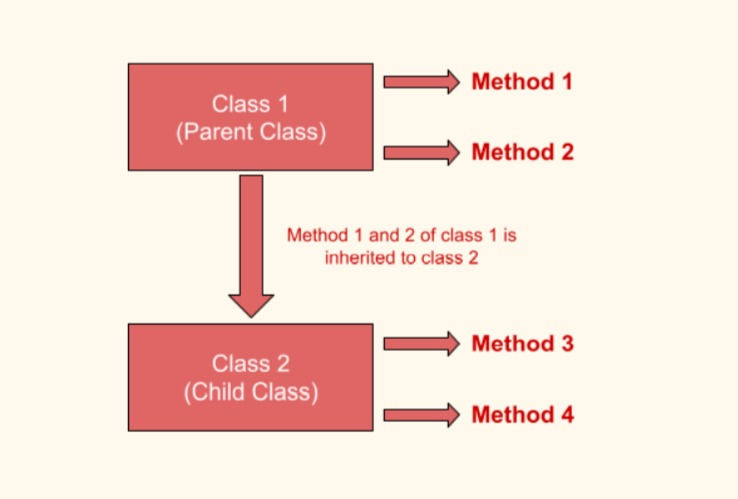
5. Encapsulation
Encapsulation helps in protecting the data in or outside of the class. It is done by using public and private data types. It helps to prevent the use and modification of some data or method in or outside of the class. Public data or methods allow access or modify it from anywhere in or outside of the class. The private data or methods can only be accessed inside the class. The private methods are represented by starting with two underscores followed by the name. Setter and getter function is used for data encapsulation in python.
Setter function:
The setter function is used to set values inside the class without directly accessing the private variable. In the example below, "setPrice" is used as a setter function to set the new price value for the computer.
Getter function:
It is the same as the setter function. The getter function is used to get the value of desired variables inside the class without directly accessing the private variable. In the example below, getPrice is used as a getter function to get the new price value for the computer.
For example:
#Encapsulation
class Computer:
def __init__(self):
self.__price = 150000
def show_price(self):
print(self.__price))
def getPrice(self):
print(self.__price))
def setPrice(self, new_price):
self.__price = new_price
#Creating object
c = Computer()
c.show_price()
#Setter function
c.setPrice(120000)
c.show_price()
#Getter function
c.getPrice(120000)
c.show_price()
[Output]
150000
120000
120000
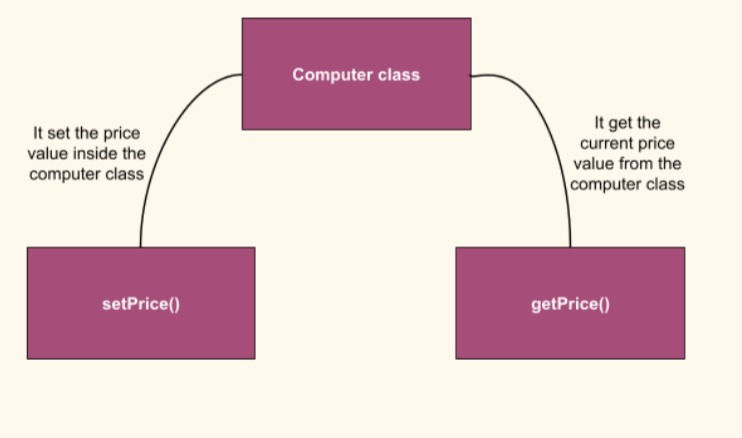
6. Polymorphism
Polymorphism is used to redefine the method with the same name from the base class. This can be performed using method overloading and method overriding.
Method overloading:
A method with the same name as the base class with a different number of arguments or data types is called method overloading.
#Parent Class
Class shape:
Def set_color(self):
return(“Red”)
#Child Class
Class circle(shape):
Def set_color(self, color=’Yellow’):
return(color)
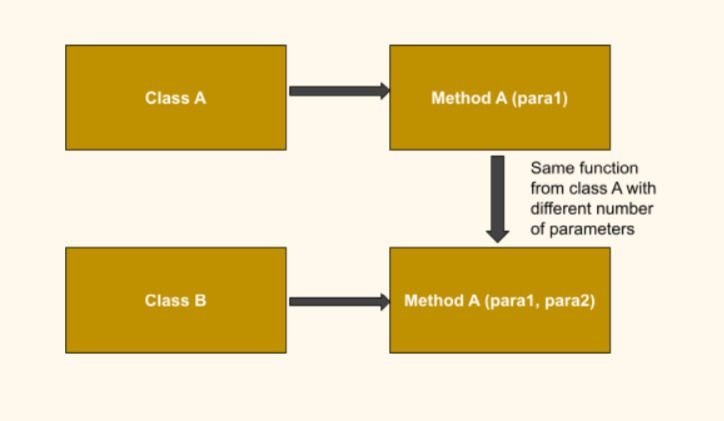
Method overriding:
A method with the same name as the base class with added extra behaviors is called method overloading.
#Parent Class
Class shape:
Def set_color(self):
return(“Red”)
#Child Class
Class circle(shape):
Def set_color(self):
return(color)
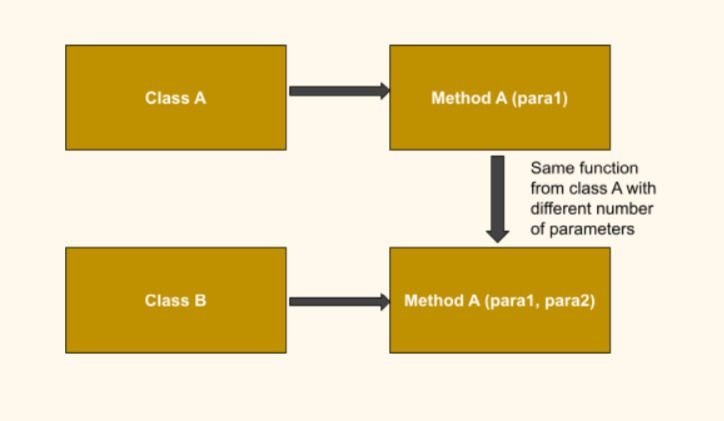
7. Data Abstraction
Data abstraction is a way to hide the sensitive or complex part of the code from the real world. It helps to protect unnecessary details of the implementation from the users. This example, shows the image_preprocessing steps are hidden from the external users. Users just need to pass the vehicle or person images to the Image_preprocessing class and output will be generated.
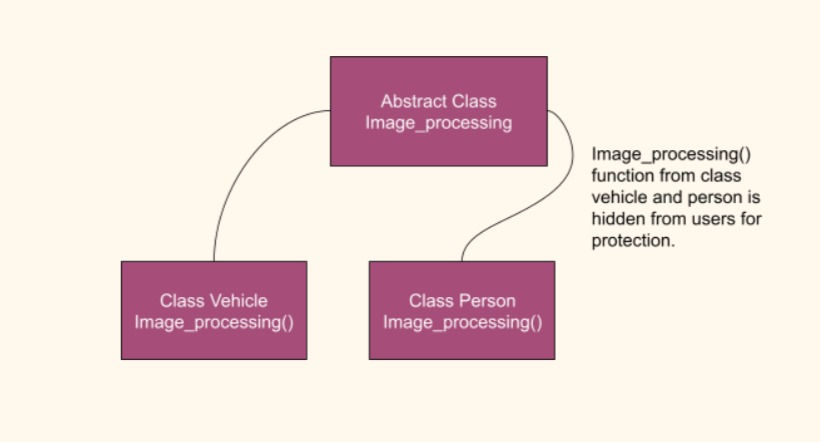
This is all about python as an object-oriented programing language. In the next blog, I will cover more on comments, docstring, modules in python.
Yorumlar